반응형
[Flutter] 플러터 기초 (3) - 플러그인 사용하기. 토스트(Toast) 예제.
Flutter 플러터 기초 (3) - 플러그인 사용하기. 토스트(Toast) 예제.
이모티콘・01・고양이 마멋 친구들 - Google Play 앱
이모티콘・01・고양이 마멋 친구들: 무료 이모티콘, 회원가입 없이! 카톡, SNS로 감정 표현이 쉬워져요. 귀여움 가득, 대화창을 더 풍성하게!
play.google.com
안녕하세요 정보처리마법사 입니다.
이번 포스팅의 주제는 Flutter 플러그인 사용하기에 관한 내용입니다.
자, 여기에서 자신에게 필요한 Packages를 검색을 해봅니다.
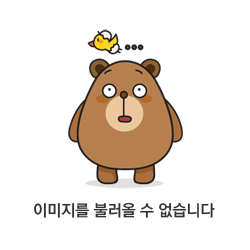
이번 포스팅에선 토스트(Toast)를 구현해보겠습니다. 😁
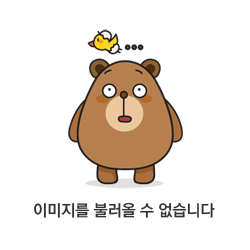
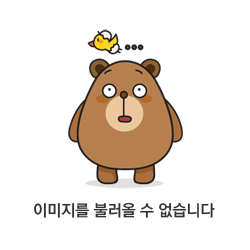
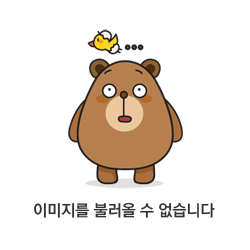
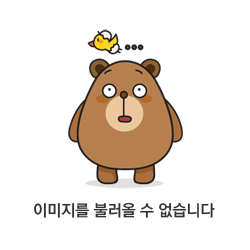
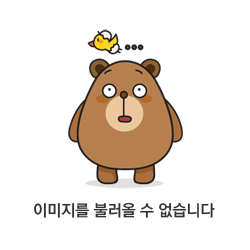
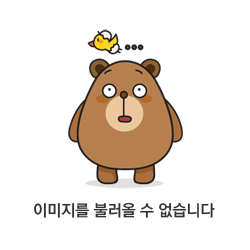
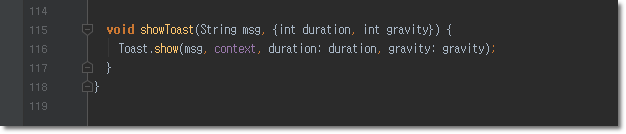
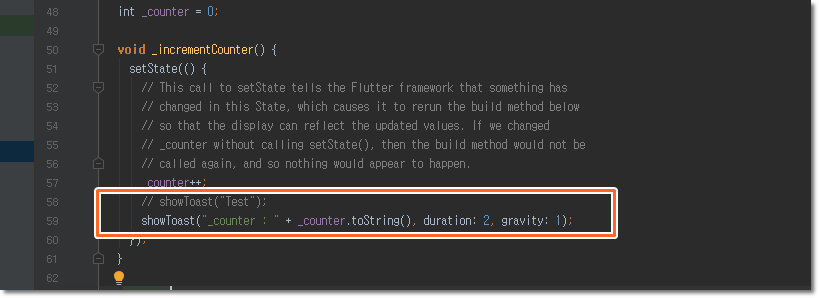
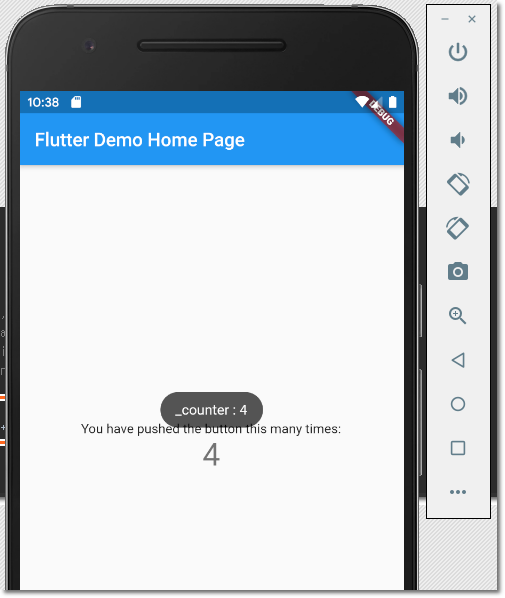
이상으로 포스팅을 마칩니다. 감사합니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
|
import 'package:flutter/material.dart';
import 'package:toast/toast.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
// This is the theme of your application.
//
// Try running your application with "flutter run". You'll see the
// application has a blue toolbar. Then, without quitting the app, try
// changing the primarySwatch below to Colors.green and then invoke
// "hot reload" (press "r" in the console where you ran "flutter run",
// or simply save your changes to "hot reload" in a Flutter IDE).
// Notice that the counter didn't reset back to zero; the application
// is not restarted.
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
// This widget is the home page of your application. It is stateful, meaning
// that it has a State object (defined below) that contains fields that affect
// how it looks.
// This class is the configuration for the state. It holds the values (in this
// case the title) provided by the parent (in this case the App widget) and
// used by the build method of the State. Fields in a Widget subclass are
// always marked "final".
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
// This call to setState tells the Flutter framework that something has
// changed in this State, which causes it to rerun the build method below
// so that the display can reflect the updated values. If we changed
// _counter without calling setState(), then the build method would not be
// called again, and so nothing would appear to happen.
_counter++;
// showToast("Test");
showToast("_counter : " + _counter.toString(), duration: 2, gravity: 1);
});
}
@override
Widget build(BuildContext context) {
// This method is rerun every time setState is called, for instance as done
// by the _incrementCounter method above.
//
// The Flutter framework has been optimized to make rerunning build methods
// fast, so that you can just rebuild anything that needs updating rather
// than having to individually change instances of widgets.
return Scaffold(
appBar: AppBar(
// Here we take the value from the MyHomePage object that was created by
title: Text(widget.title),
),
body: Center(
// Center is a layout widget. It takes a single child and positions it
// in the middle of the parent.
child: Column(
// Column is also layout widget. It takes a list of children and
// arranges them vertically. By default, it sizes itself to fit its
// children horizontally, and tries to be as tall as its parent.
//
// Invoke "debug painting" (press "p" in the console, choose the
// "Toggle Debug Paint" action from the Flutter Inspector in Android
// Studio, or the "Toggle Debug Paint" command in Visual Studio Code)
// to see the wireframe for each widget.
//
// Column has various properties to control how it sizes itself and
// how it positions its children. Here we use mainAxisAlignment to
// center the children vertically; the main axis here is the vertical
// axis because Columns are vertical (the cross axis would be
// horizontal).
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
), // This trailing comma makes auto-formatting nicer for build methods.
);
}
void showToast(String msg, {int duration, int gravity}) {
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4f; text-decoration:none">Colored by Color Scripter
|
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none; color:white">cs |
Ctrl + C , Ctrl + V
이상으로 포스팅을 마칩니다. 감사합니다.
잘 못 된 정보가 있으면 말씀해주세요.
공감버튼 클릭은 작성자에게 큰 힘이 됩니다. 행복한 하루 되세요.

“파트너스 활동을 통해 일정액의 수수료를 제공받을 수 있음"
반응형
'Flutter' 카테고리의 다른 글
[Flutter] 플러터 기초 (6) - 리스트뷰 위젯(ListView Widget) 예제. (0) | 2019.07.10 |
---|---|
[Flutter] 플러터 기초 (5) - cupertino ui button, material ui button (0) | 2019.06.28 |
[Flutter] 플러터 기초 (4) - Hello World, FloatingActionButton 등 기본 예제. (0) | 2019.06.28 |
[Flutter] 플러터 기초 (2) - 리스트, 스낵바 등 기초 연습. (3) | 2019.06.26 |
[Flutter] 플러터 기초 (1) - 초반 셋팅 (안드로이드스튜디오 사용) (2) | 2019.06.25 |